Recently, I have been working on a MERN project to manage a vehicle fleet. While creating the vehicle landing pages, I realized that there was currently no way to change the order of the vehicle images except for manually modifying the database entries. Thinking this should be a quick fix, I started modifying the edit vehicle page to add this capability.
I tried to brute force the image re-ordering functionality using react-beautiful-dnd with the help of GitHub Copilot. I think I was running into some compatibility issues as I was running React 18. This post (Is this support in React 18? · Issue #2399 · atlassian/react-beautiful-dnd (github.com)) mentions that some users were able to get it running by turning StrictMode off, but since I am new to React, I wanted StrictMode on to help highlight problems with my code. So, I then moved on to try the solution user GiovanniACamacho mentions which is to render the Droppable items inside an animation frame. This has worked for a few users, but I did not have luck with this – possibly, as I was on the hunt for a quick solution.
At this point, I switched to a react-dnd and react-dnd-html5-backend solution. The first time I tried this, I had issues with the actual movement of the image order values in the vehicleImages object. The vehicleImages object in my case was structured as follows:
{
"imageURL": "imageURL",
"imageOrder": "imageOrder"
}
At this point, I was about to give up on the drag-and-drop method to re-order images and move to something I was more familiar with – a checkbox beside each image that allowed you to select the order of that given image. I actually started implementing this and mid-way realized that this would also be annoying to implement as I would have to come up with a method to update the other imageOrder values. Not only this, I would end up with a user experience that I personally wouldn’t like.
So, I switched back to implementing the drag-and-drop functionality. This time with more strategy than brute-force with the help of GitHub Copilot – I created an empty React project to allow me to just experiment with drag-and-drop methods.
To setup my environment, I created an object with a similar structure to what I desired in the vehicle fleet management project. Once I had the structure and placeholder images in place, I began creating the drag-and-drop functionality using react-dnd and react-dnd-html5-backend. With this, I created a Card object for each image – this was done to allow me to display the current order number for the given image. I also implemented this to have a “Save button” that commits the order for the images rather than auto-saving, though both should be possible.
The implemented React image sorting project can be found on my GitHub: utkarshsaini/react-dnd-image-sorter: A react project to experiment with drag-and-drop functionality for an image sorter (github.com).
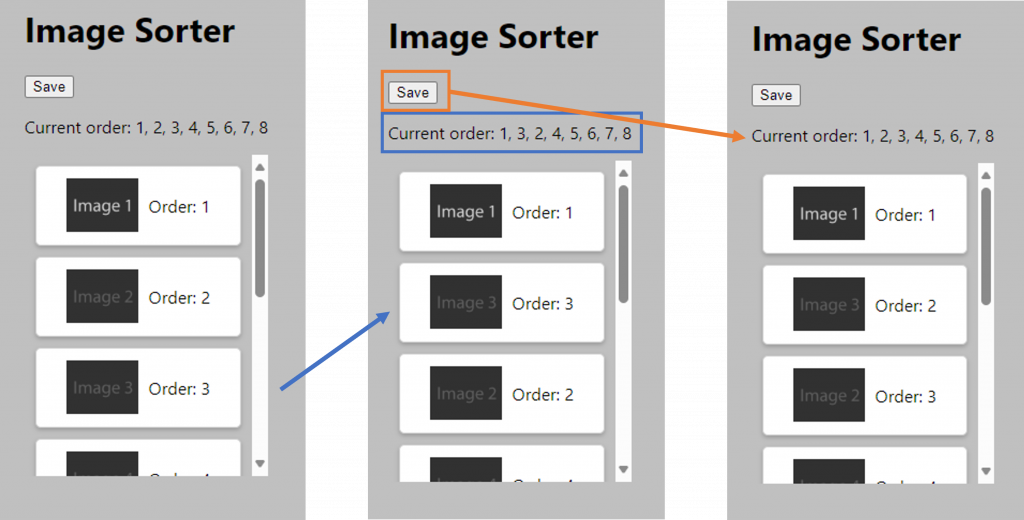
I am currently working to integrate this implementation into the vehicle fleet management project I am working on.